Lambda expression: - A Lambda expression is an anonymous function that can contain expressions and statements, and can be used to create delegates or expression tree types.
In simple words Lambda expression is nothing but it is an extended version of delegates or in other words it making delegates simpler.
All Lambda expression uses the lambda operator (=>).
The left side of the lambda operator specifies the input parameters (if any) and the right side hold the expression or statement block.
Let’s create a simple example to understand the concept of Lambda expression in much better manner.
In order to see it practically you just need to follow the following steps.
Step1: - Create a simple Console Application for that just open Visual Studio >> go to >> File >> New >> Project >> Windows >> Select Console Application.
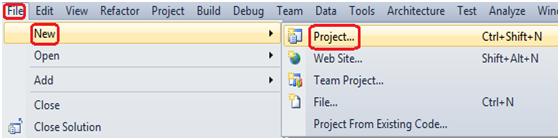

Step2: - Now, simply just add the below code snippet in to program.cs file of your Console Application.
Now, simply just run your console application and you should see the result as shown in the below diagram.
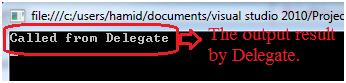
The above code is nice it work’s properly but the concern here is the code complexity; you can see that for a simple line of output we have created extra function, which makes your code little lengthy and not very easy to read.
So Lambda Expression helps us to solve the above problem in simplified manner and making our code more readable and understandable.
Let’s see how exactly Lambda Expression help to solve the above problem for that you just need to follow the following step.
Step3: - Now, just simply modify the program.cs file like below code snippet.
Now, simply just run your console application and will see result like below output diagram.
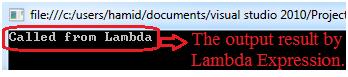
Watch the following interesting video on threading in C#.NET: -
Get our more important Dotnet interview questions materials.
Regards,
Visit more author's other Most asked Dotnet interview question
In simple words Lambda expression is nothing but it is an extended version of delegates or in other words it making delegates simpler.
All Lambda expression uses the lambda operator (=>).
The left side of the lambda operator specifies the input parameters (if any) and the right side hold the expression or statement block.
Let’s create a simple example to understand the concept of Lambda expression in much better manner.
In order to see it practically you just need to follow the following steps.
Step1: - Create a simple Console Application for that just open Visual Studio >> go to >> File >> New >> Project >> Windows >> Select Console Application.
Step2: - Now, simply just add the below code snippet in to program.cs file of your Console Application.
class Program { //created a delegate. delegate void PointToCallMe(string str); static void Main(string[] args) { //Delegate,which points to the CallMe Function. PointToCallMe objDelegate = new PointToCallMe(CallMe); objDelegate.Invoke("Called from Delegate"); Console.ReadLine(); } public static void CallMe(string str) { Console.WriteLine(str); } }In the above code snippet you can see that I have created a function CallMe with a string parameter “str” and I have created a delegate which points toward the CallMe function.
Now, simply just run your console application and you should see the result as shown in the below diagram.
The above code is nice it work’s properly but the concern here is the code complexity; you can see that for a simple line of output we have created extra function, which makes your code little lengthy and not very easy to read.
So Lambda Expression helps us to solve the above problem in simplified manner and making our code more readable and understandable.
Let’s see how exactly Lambda Expression help to solve the above problem for that you just need to follow the following step.
Step3: - Now, just simply modify the program.cs file like below code snippet.
class Program { //created a delegate. delegate void PointToCallMe(string str); static void Main(string[] args) { //Lambda Expression. PointToCallMe objLambda = str => Console.WriteLine(str); objLambda.Invoke("Called from Lambda"); Console.ReadLine(); } }Now, in the above code snippet you can see that I have just eliminated the extra created CallMe function and just written a few line of Lambda Expression, which makes your code more readable and understandable with a few line of code.
Now, simply just run your console application and will see result like below output diagram.
Watch the following interesting video on threading in C#.NET: -
Get our more important Dotnet interview questions materials.
Regards,
Visit more author's other Most asked Dotnet interview question
No comments:
Post a Comment