Tracing: - Tracing is a way to monitor the execution of yourASP.NET application. You can record exception details and program flow in a way that doesn't affect the program's output.
In simple words when you monitor your application during production or deployment phase is called Tracing.
Tracing can be done in the following ways.
1. Page level: - Enable tracing at page level display can be seen on browser.
2. Web.Config level: - Enable Tracing throughout the application.
Let’s see a simple example on how exactly we can achieve Tracing in your application.
In order to do tracing just follow the following steps.
Step1: - create an ASP.NET Web Application for that just Go To > File >
New > Project > Web > ASP.NET Empty Web Application.
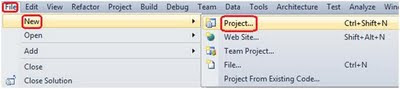

Now just add a form to the application for that just right click on the application > Add > Add New Item > Select Web Form.
Add three buttons on the WebForm.aspx page like below diagram.
Step2: - Add the below code snippet in WebForm1.aspx.cs page.
Step3: - Now let’s see how you can achieve Page level Tracing.
ASP.NET tracing can be enabled on a page-by-page basis by adding "Trace=true" to the Page directive in any ASP.NET page:
Additionally, you can add the TraceMode attribute that sets SortByCategory or the default, SortByTime. You can use SortByTime to see the methods that take up the most CPU time for your application. You can enable tracing programmatically using the Trace.IsEnabled property.
Now, just run your application you will see output like below diagram.
Step4: - Now let’s see that how you can achieve Web.Config level Tracing.
You can enable tracing for the entire application by adding tracing settings in
web.config. In below example, pageOutput="false" and requestLimit="20"
are used, so trace information is stored for 20 requests, but not displayed on
the page because pageOutput attribute is set to false.
Now what happened is all of your application pages are now enabled to tracing.
Step5: - Now let’s see what Trace.axd means.
Trace.axd: - Page output of tracing shows only the data collected for the current page request. However, if you want to collect detailed information for all the requests then we need to use Trace.axd. We can invoke Trace.axd tool for the application using the following URL http://localhost/application-name/trace.axd. Simply replace page name in URL with Trace.axd. That is, in our case. We should use following URL (Address bar) for our application as shown below.
Let’s see a simple demonstration on Trace.axd.
Just run your application and click on any one of the button and later in the url bar just replace the name like http://localhost1348/Trace.axd , will see the output like below diagram.
Now just click on the last view details and will see the tracing details like below diagram.
Also view the following video on ASP.NET Web.config transformation: -
Also see our tutorials on ASP.NET interview questions
Regards,
View more author’s blog on ASP.NET interview questions
In simple words when you monitor your application during production or deployment phase is called Tracing.
Tracing can be done in the following ways.
1. Page level: - Enable tracing at page level display can be seen on browser.
2. Web.Config level: - Enable Tracing throughout the application.
Let’s see a simple example on how exactly we can achieve Tracing in your application.
In order to do tracing just follow the following steps.
Step1: - create an ASP.NET Web Application for that just Go To > File >
New > Project > Web > ASP.NET Empty Web Application.
Now just add a form to the application for that just right click on the application > Add > Add New Item > Select Web Form.
Add three buttons on the WebForm.aspx page like below diagram.
Step2: - Add the below code snippet in WebForm1.aspx.cs page.
using System.Diagnostics; namespace TracingApplication { public partial class WebForm1 : System.Web.UI.Page { Random obj = new Random();// created random object int intRandom = 0; protected void Page_Load(object sender, EventArgs e) { intRandom = obj.Next(5);// initialized value to the intRandom variable. } protected void Button1_Click(object sender, EventArgs e) { Trace.Warn("Button1 has been clicked"); } protected void Button2_Click(object sender, EventArgs e) { Trace.Warn("Button2 has been clicked"); } protected void Button3_Click(object sender, EventArgs e) { Trace.Warn("Button3 has been clicked"); } } }
Step3: - Now let’s see how you can achieve Page level Tracing.
ASP.NET tracing can be enabled on a page-by-page basis by adding "Trace=true" to the Page directive in any ASP.NET page:
<%@ Page Language="C#" Trace="true" AutoEventWireup="true" CodeBehind="WebForm1.aspx.cs" Inherits="TracingApplication.WebForm1" %>
Additionally, you can add the TraceMode attribute that sets SortByCategory or the default, SortByTime. You can use SortByTime to see the methods that take up the most CPU time for your application. You can enable tracing programmatically using the Trace.IsEnabled property.
Now, just run your application you will see output like below diagram.
Step4: - Now let’s see that how you can achieve Web.Config level Tracing.
You can enable tracing for the entire application by adding tracing settings in
web.config. In below example, pageOutput="false" and requestLimit="20"
are used, so trace information is stored for 20 requests, but not displayed on
the page because pageOutput attribute is set to false.
<configuration> <appSettings/> <connectionStrings/> <system.web> <compilation debug="false" /> <authentication mode="Windows" /> <trace enabled ="true" pageOutput ="false" requestLimit ="20" traceMode ="SortByTime " /> </system.web> </configuration>
Now what happened is all of your application pages are now enabled to tracing.
Step5: - Now let’s see what Trace.axd means.
Trace.axd: - Page output of tracing shows only the data collected for the current page request. However, if you want to collect detailed information for all the requests then we need to use Trace.axd. We can invoke Trace.axd tool for the application using the following URL http://localhost/application-name/trace.axd. Simply replace page name in URL with Trace.axd. That is, in our case. We should use following URL (Address bar) for our application as shown below.
Let’s see a simple demonstration on Trace.axd.
Just run your application and click on any one of the button and later in the url bar just replace the name like http://localhost1348/Trace.axd , will see the output like below diagram.
Now just click on the last view details and will see the tracing details like below diagram.
Also view the following video on ASP.NET Web.config transformation: -
Also see our tutorials on ASP.NET interview questions
Regards,
View more author’s blog on ASP.NET interview questions